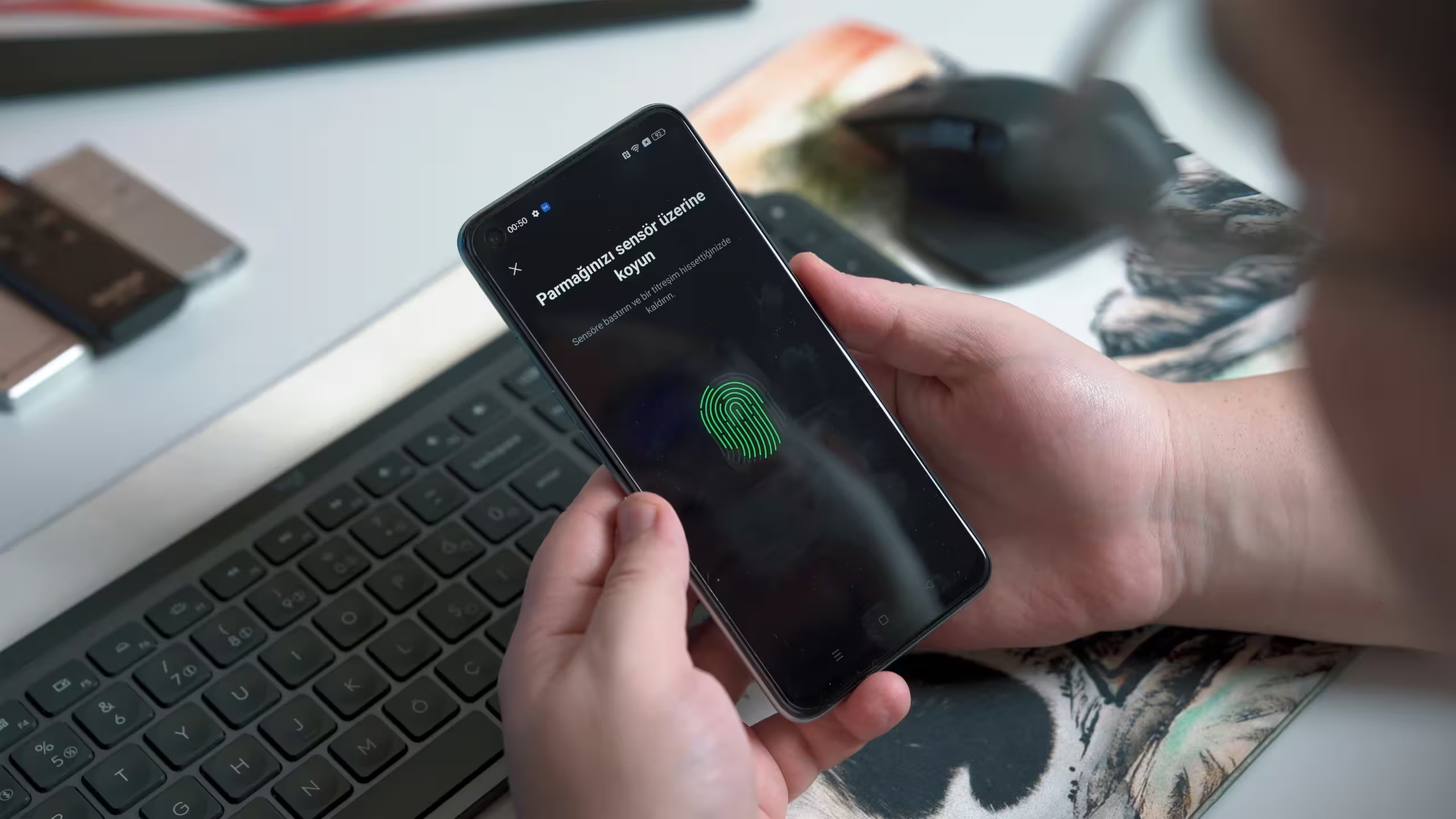
How to use OAuth
Published: March 21, 2025
Modern web applications often allow users to log in using third-party services like Google, Facebook, etc. This type of authentication is powered by OAuth 2.0. In this blog post, we’ll explain what OAuth 2.0 is and how to implement Google login in a Laravel application using the Laravel Socialite package.
🔐 What is OAuth 2.0 and Why Use It?
OAuth 2.0 is a standard protocol for authorization that allows apps to access user data from another service without needing the user’s password. Here’s why it’s beneficial:
- Security: Users don’t enter passwords into your app — they log in via Google.
- Verified Emails: You receive real, verified emails from Google.
- Better UX: One-click login and registration.
- Standardized: Widely supported and easy to integrate.
Bonus: Google uses OpenID Connect (built on top of OAuth) to securely verify identity.
🚀 Implementation: Google OAuth Login in Laravel
We’ll use Laravel Socialite to integrate Google login. Let’s walk through the process step-by-step.
1. Install Laravel Socialite
composer require laravel/socialite
2. Create Google OAuth Credentials
- Go to the Google Cloud Console.
- Create a new project or select an existing one.
- Navigate to APIs & Services > OAuth consent screen.
- Choose “External”.
- Fill in app info (name, support email, etc.).
- Go to Credentials > Create Credentials > OAuth client ID.
- Choose Web Application.
- Set Authorized redirect URI to:
http://localhost:8000/auth/google/callback
- Copy your Client ID and Client Secret.
3. Configure Laravel
In your .env
file:
GOOGLE_CLIENT_ID=your-client-id
GOOGLE_CLIENT_SECRET=your-client-secret
GOOGLE_REDIRECT_URI=http://localhost:8000/auth/google/callback
In config/services.php
:
'google' => [
'client_id' => env('GOOGLE_CLIENT_ID'),
'client_secret' => env('GOOGLE_CLIENT_SECRET'),
'redirect' => env('GOOGLE_REDIRECT_URI'),
],
4. Add Routes
In routes/web.php
:
use App\Http\Controllers\GoogleLoginController;
Route::get('/auth/google', [GoogleLoginController::class, 'redirectToGoogle'])->name('google.redirect');
Route::get('/auth/google/callback', [GoogleLoginController::class, 'handleGoogleCallback'])->name('google.callback');
5. Create Controller
php artisan make:controller GoogleLoginController
In GoogleLoginController.php
:
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Support\Facades\Auth;
use Laravel\Socialite\Facades\Socialite;
use Illuminate\Support\Str;
class GoogleLoginController extends Controller
{
public function redirectToGoogle()
{
return Socialite::driver('google')->redirect();
}
public function handleGoogleCallback()
{
$googleUser = Socialite::driver('google')->stateless()->user();
$user = User::updateOrCreate(
[ 'email' => $googleUser->getEmail() ],
[
'name' => $googleUser->getName(),
'password' => bcrypt(Str::random(24))
]
);
Auth::login($user);
return redirect()->route('home')->with('success', 'Successfully logged in!');
}
}
6. Add Google Login Button
In your Blade view:
<a href="{{ route('google.redirect') }}" class="btn btn-danger">
<i class="fab fa-google me-2"></i> Sign in with Google
</a>
⚠️ Security Tips
- Always use HTTPS in production.
- Never commit
.env
files with secrets. - Validate user data even if it comes from Google.
- Keep Laravel and Socialite up to date.
- Avoid using
->stateless()
unless necessary for API-only apps.
✅ Summary
Google OAuth lets users log in without forms or passwords. Laravel Socialite makes it easy to integrate. After login, your app still manages user accounts — Google is only used to verify identity.
OAuth improves security, boosts UX, and simplifies onboarding. Give your users the experience they expect — secure, fast, and convenient sign-ins.
Let me know if you’d like to expand this into a full article with GitHub repo and video walkthrough!
Modern web applications often allow users to log in using third-party services like Google, Facebook, etc. This type of authentication is powered by OAuth 2.0. In this blog post, we’ll explain what OAuth 2.0 is and how to implement Google login in a Laravel application using the Laravel Socialite package.
🔐 What is OAuth 2.0 and Why Use It?
OAuth 2.0 is a standard protocol for authorization that allows apps to access user data from another service without needing the user’s password. Here’s why it’s beneficial:
- Security: Users don’t enter passwords into your app — they log in via Google.
- Verified Emails: You receive real, verified emails from Google.
- Better UX: One-click login and registration.
- Standardized: Widely supported and easy to integrate.
Bonus: Google uses OpenID Connect (built on top of OAuth) to securely verify identity.
🚀 Implementation: Google OAuth Login in Laravel
We’ll use Laravel Socialite to integrate Google login. Let’s walk through the process step-by-step.
1. Install Laravel Socialite
composer require laravel/socialite
2. Create Google OAuth Credentials
- Go to the Google Cloud Console.
- Create a new project or select an existing one.
- Navigate to APIs & Services > OAuth consent screen.
- Choose “External”.
- Fill in app info (name, support email, etc.).
- Go to Credentials > Create Credentials > OAuth client ID.
- Choose Web Application.
- Set Authorized redirect URI to:
http://localhost:8000/auth/google/callback
- Copy your Client ID and Client Secret.
3. Configure Laravel
In your .env
file:
GOOGLE_CLIENT_ID=your-client-id
GOOGLE_CLIENT_SECRET=your-client-secret
GOOGLE_REDIRECT_URI=http://localhost:8000/auth/google/callback
In config/services.php
:
'google' => [
'client_id' => env('GOOGLE_CLIENT_ID'),
'client_secret' => env('GOOGLE_CLIENT_SECRET'),
'redirect' => env('GOOGLE_REDIRECT_URI'),
],
4. Add Routes
In routes/web.php
:
use App\Http\Controllers\GoogleLoginController;
Route::get('/auth/google', [GoogleLoginController::class, 'redirectToGoogle'])->name('google.redirect');
Route::get('/auth/google/callback', [GoogleLoginController::class, 'handleGoogleCallback'])->name('google.callback');
5. Create Controller
php artisan make:controller GoogleLoginController
In GoogleLoginController.php
:
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Support\Facades\Auth;
use Laravel\Socialite\Facades\Socialite;
use Illuminate\Support\Str;
class GoogleLoginController extends Controller
{
public function redirectToGoogle()
{
return Socialite::driver('google')->redirect();
}
public function handleGoogleCallback()
{
$googleUser = Socialite::driver('google')->stateless()->user();
$user = User::updateOrCreate(
[ 'email' => $googleUser->getEmail() ],
[
'name' => $googleUser->getName(),
'password' => bcrypt(Str::random(24))
]
);
Auth::login($user);
return redirect()->route('home')->with('success', 'Successfully logged in!');
}
}
6. Add Google Login Button
In your Blade view:
<a href="{{ route('google.redirect') }}" class="btn btn-danger">
<i class="fab fa-google me-2"></i> Sign in with Google
</a>
⚠️ Security Tips
- Always use HTTPS in production.
- Never commit
.env
files with secrets. - Validate user data even if it comes from Google.
- Keep Laravel and Socialite up to date.
- Avoid using
->stateless()
unless necessary for API-only apps.
✅ Summary
Google OAuth lets users log in without forms or passwords. Laravel Socialite makes it easy to integrate. After login, your app still manages user accounts — Google is only used to verify identity.
OAuth improves security, boosts UX, and simplifies onboarding. Give your users the experience they expect — secure, fast, and convenient sign-ins.
Let me know if you’d like to expand this into a full article with GitHub repo and video walkthrough!
New web is waiting for you
We turn your website visitors into euros. Contact us and increase your profits today!